By Lim Yi Jie
About the project
Our team of 5 members were tasked to either enhance or morph an existing command line interface desktop address book application for our Software Engineering project. We chose to morph the original application into a group scheduling application called TimeBook. TimeBook aims to help busy NUS undergraduates keep track of the schedules of the user and his or her friends, and aid the user to arrange meetings with his or her friends.
This is a screenshot of our application.
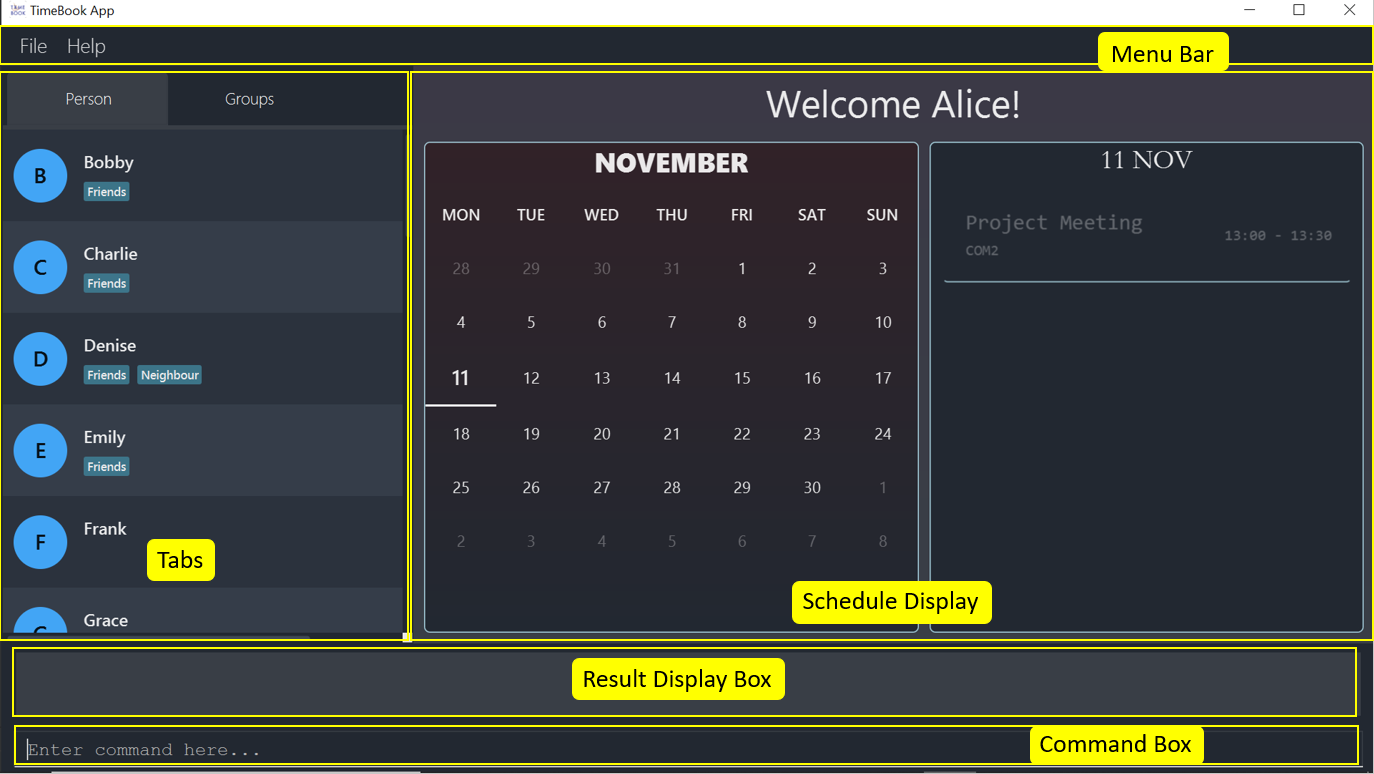
Figure 1. GUI for TimeBook
My role was to design and write codes to display the combined schedules of NUS undergraduates from a group in the GUI elegantly and intuitively. In the following sections, my contribution and the relevant documentations that I have made will be elaborated in more detail.
Note the common symbols and formatting used in this document.
-
— Important information.
-
show
— Monospaced uncapitalised word indicates that this is a command that can be executed in the command line. -
ScheduleView
— Monospace capitalised indicates that this is a class or component in TimeBook.
Overview of contributions
This section provides an overview of the code, documentation and administrative contributions to the team project
-
Code contributed: Please take a look here to see the codes that I have contributed.
Main feature added: The schedule graphic of multiple schedules in TimeBook.
-
What: Enables users to see a 7-day schedule graphic in the form of a time table in TimeBook. The
show
andexport
command does this. -
Justification: This is the key feature of TimeBook as it directly addresses the problem of having to tediously cross reference every separate schedule in order to identify free time slots for group meetings.
-
Highlights: This feature that I have implemented fits well with the other features that my teammates developed. For example, one of my teammates developed an NUSMods parser that makes use of parsing NUSMods links into data that is then displayed using my feature. Implementing this feature is not easy as I had to first create an empty time table and figure out a way to add events (represented by blocks) into this empty time table without distorting the other contents in the time table. In addition, I had to design the graphic such that resizing the application or adjusting the screen resolution will not distort the time table. Finally, I had to test my feature using TestFX to make sure that the graphic rendered is correct.
-
Credits: TestFX for providing a framework for automated GUI testing.
Additional features that enhances the schedule graphic shown in TimeBook.
-
Toggle the schedule graphic to show the graphics for subsequent week’s schedule. This is done through the
togglenext
command.-
Enhanced usability of our application.
-
-
Look at only schedules belonging to some members of a group. This is done through the
lookat
command.-
Implemented in order for users to filter and inspect the schedules of some group members in a particular group.
-
Other contributions:
Contributions to User Guide
Our team had to override the original addressbook documentation with the instructions for TimeBook. This section shows my contributions to the User Guide and my ability to convey instructions for the application to target users.
Show group: show
Want to see your group’s schedules to plan for your next group meeting? The show
command is what you need to see
the schedules of your group members! You can view a group’s schedule up to 4 weeks in advance.
Format: show g/GROUP_NAME
Examples:
-
Let’s say you want to organise a group meeting for a group named CS2103. Simply enter
show g/CS2103
in the command line as shown belowFigure 1. Command box -
Hit the Enter key and you should see the details of this group in a similar window below.
Figure 2. Result after executingshow g/CS2103
Look at some members: lookat
Suppose that all of your group members are extremely busy and the common free time is too short to do any productive work,
you may use the lookat
command to see if you can meet with some of your group members. You must be viewing a group’s
schedule in order for this command to work.
Format:
-
lookat [n/Name]…
The lookat
command takes in any number of n/Name
arguments and highlights the group members according to name.
Example:
Let’s use the same schedule exactly like the one shown above.
-
Type
lookat n/Bobby n/Denise
if you are interested to inspect both Bobby’s and Denise’s schedules! You should see that both of these schedules become highlighted.
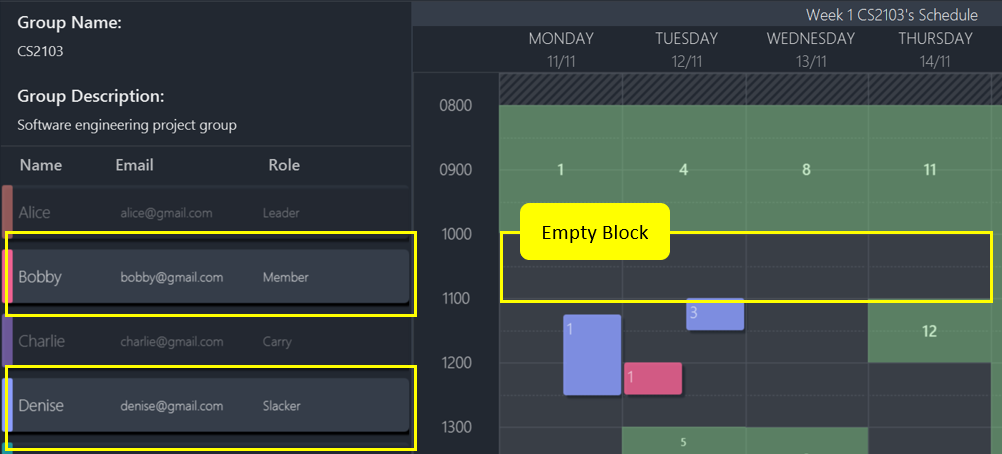
Contributions to Developer Guide
This section shows my contributions to the developer guide and my ability to convey technical information for my feature in the application that my team has developed.
Visual Representation of individual’s or group’s schedule
Visual representation here refers to the graphics you see when you view a group or an individual’s schedule in TimeBook. We will first describe how the graphics are created.
All of these graphics are created in the ScheduleView
class. The object oriented domain model below illustrates the problem domain of the ScheduleView
class in TimeBook.
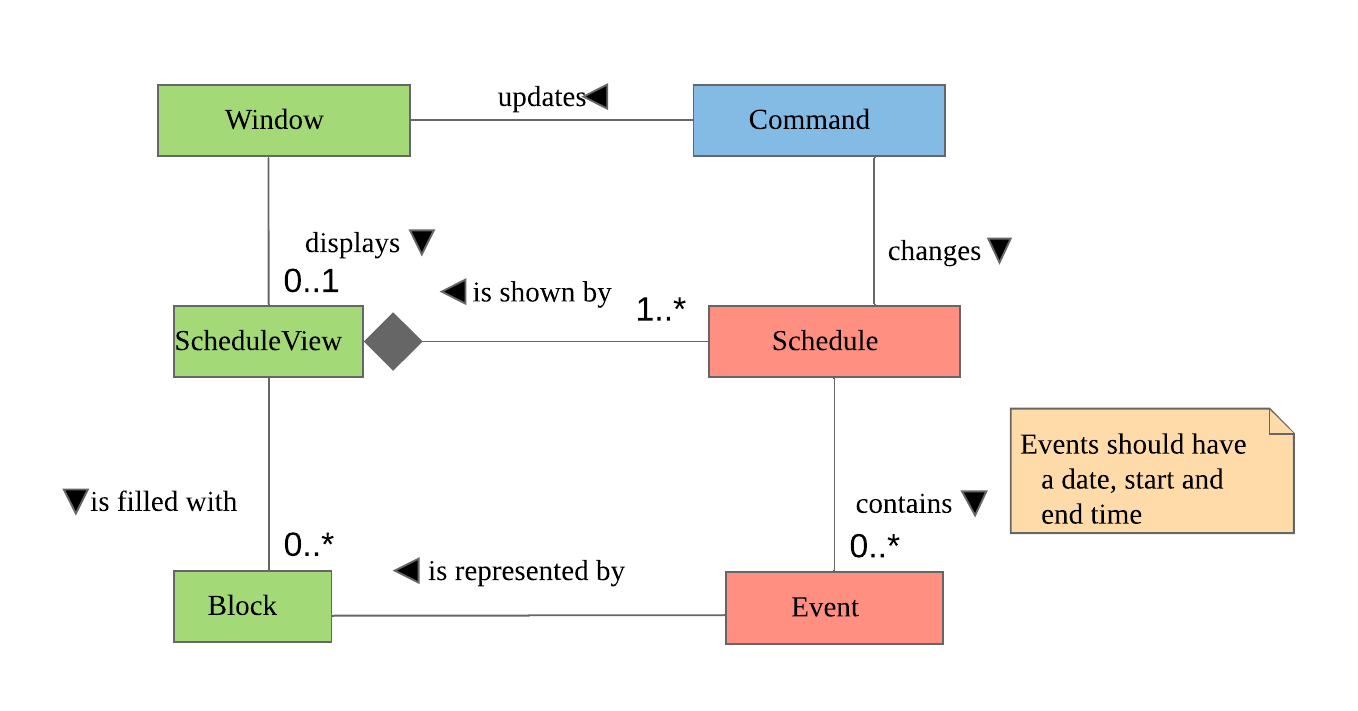
ScheduleView
.The ScheduleView
class in TimeBook follows the above model closely. Let’s walk you through how the graphics are created.
-
Following the model, we have a class
PersonTimeslot
that behaves like an event time slot. EachPersonTimeslot
object thus have a date, a start time and an end time. -
Separate the given
PersonTimeslot
objects into lists by dates and sort the time slots according to start times. Each list acts as aSchedule
for a particular date. -
For each date, create a VBox (a container to to stack
Block
objects vertically). Eventually, each VBox will contain all the time slot blocks for the a particular date.-
Condition: If the first
PersonTimeslot
in the list starts after 8am (TimeBook’s schedule start time), stack an emptyBlock
in the VBox with the same height as the duration between 8am and the start time of thisPersonTimeslot
object to represent the initial offset region.
-
-
Loop through each
PersonTimeslot
object in the list, stack a colouredBlock
in the same VBox. Each of theBlock
should have the same height as the duration between the start and end time of its correspondingPersonTimeslot
object. -
Stack in empty
Block
to fill the gaps between the end time of the currentPersonTimeslot
and the start time of the nextPersonTimeslot
in the list.
Now that you have seen how the graphics for TimeBook are created, the next step would be to control what graphics to show. As such,
we made use of an abstract class ScheduleViewManager
to control the creation of ScheduleView
objects.
The two classes that extend from ScheduleViewManager
are IndividualScheduleViewManager
and GroupScheduleViewManager
.
The following methods are implemented in ScheduleViewManager
to control the schedules displayed in the window.
-
ScheduleViewManager#getInstanceOf(ScheduleDisplay)
— Instantiates theScheduleViewManager
with a givenScheduleDisplay
object. TheScheduleDisplay
object contains all the information needed to generate a schedule view. -
ScheduleViewManager#scrollNext()
— Scrolls the schedule shown down. Once it reaches the bottom, it will start back at the top. -
ScheduleViewManager#toggleNext()
— Modifies the schedule shown to show the next week’s schedule. The schedule shown can at most show up to 4 weeks in advance. Once the fourth week is reached, it will start back at the first week. -
ScheduleViewManager#filterPerson(List<Name>)
Filters the schedule shown to the given list of names. This method only works when the schedule shown belongs to group.
A sample usage of the ScheduleViewManager is described below.
Step 1. The user wants to view a group called "Three musketeers" consisting of 3 members, Alice, Ben and Carl in TimeBook and executes the command
show g/Three musketeers
in the command line. The state of ScheduleViewManager
will be initialised to show only the group’s schedule for the first week as shown in the object diagram below.
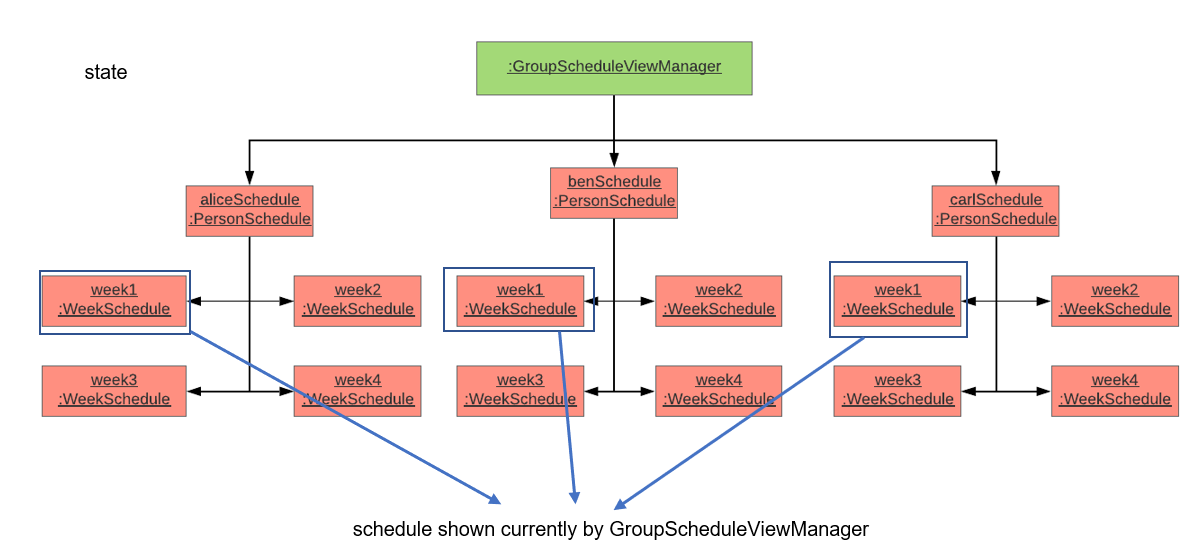
show
command is executed.Step 2. Suppose the user thinks that arranging a group meeting on the first week is too rushed, so he executes the togglenext
command to view the group’s schedule for the next week.
The state of ScheduleViewManager
is then modified to show the second week of the group’s schedule as shown in the diagram below.
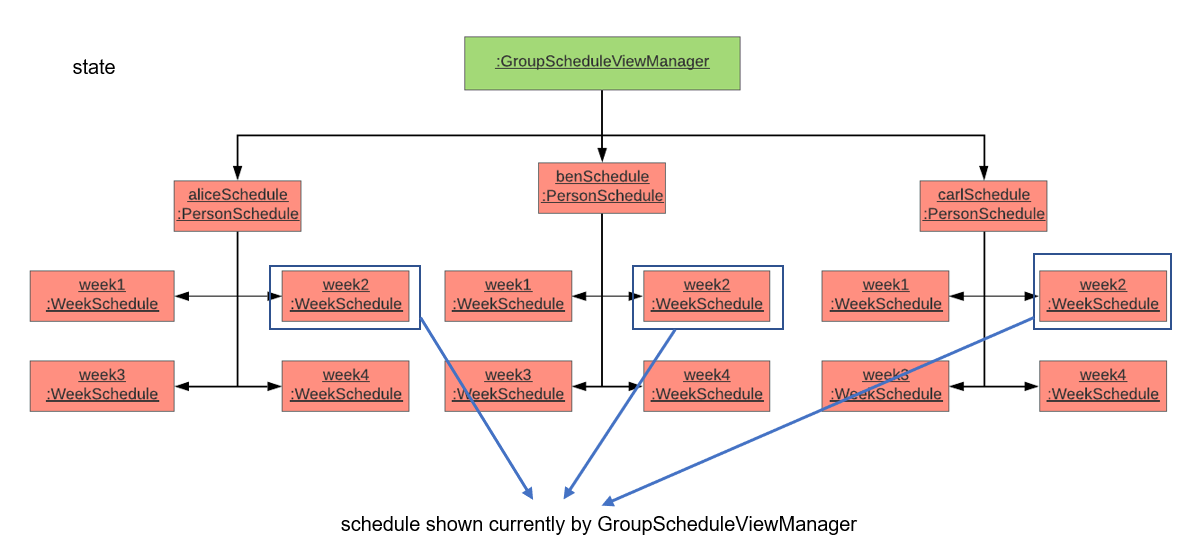
togglenext
command is executed.Step 3. Suppose the user now wants to inspect some of his group members' schedules, and he executes the lookat
command to inspect Alice’s and Carl’s schedules.
The state of ScheduleViewManager
is once again modified to only show the specified group members' schedules in the object diagram below.
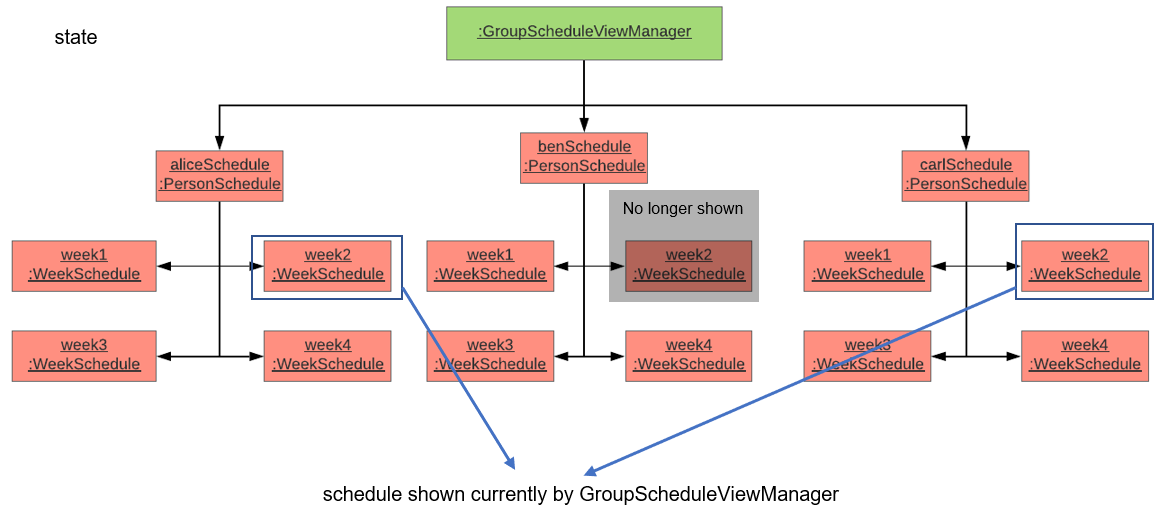
lookat
command is executed.Now that we have the full picture of how the graphics are created and controlled, we are ready to show how the user obtain a visual representation of a person or group’s schedule using the show
command.
The following sequence diagram shows the sequence of events that lead to changes in the UI when an example of the show
command is executed for a group called CS2103.
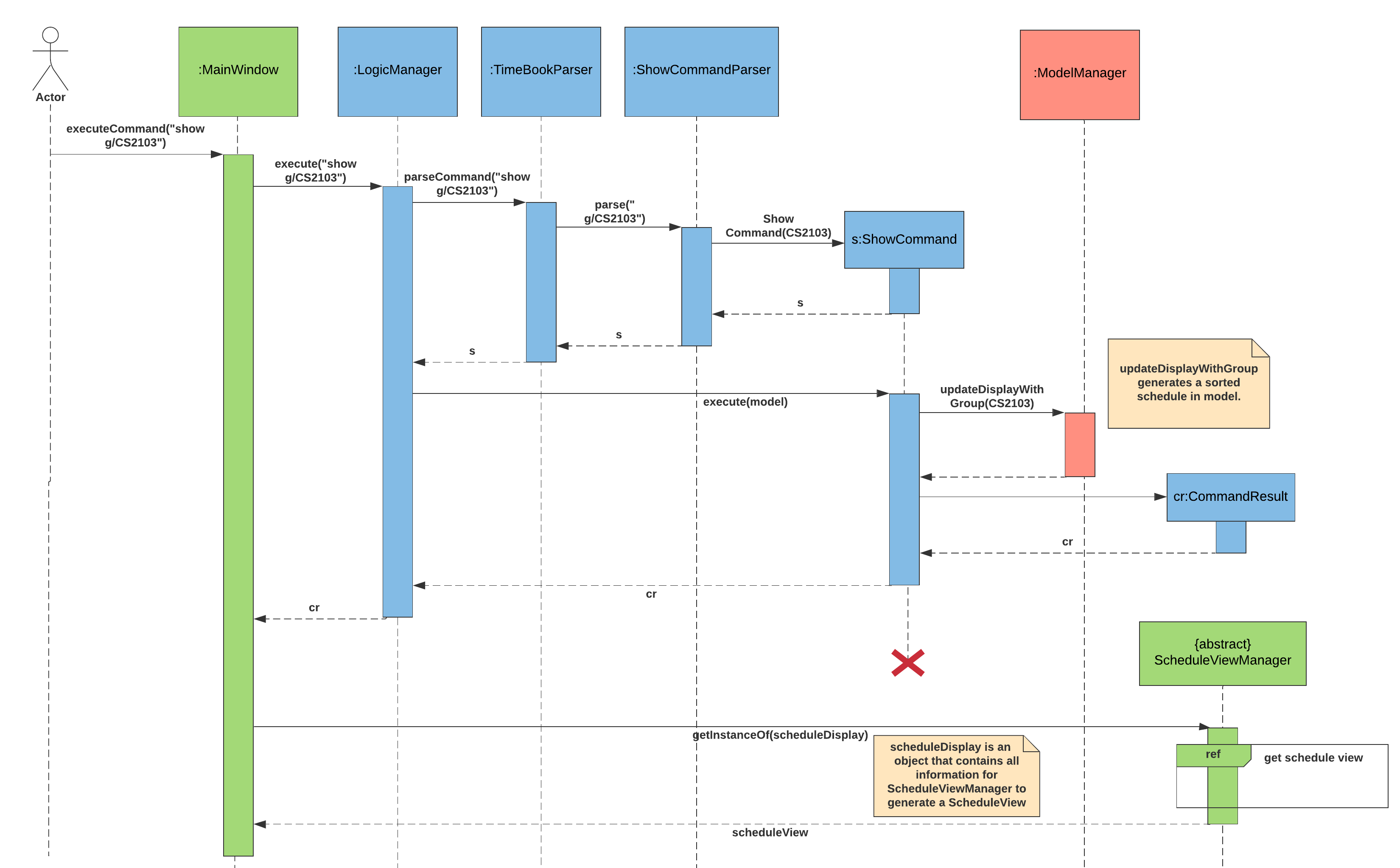
show
command.In order to make the diagram look less messy, a reference diagram shown below is created to show what happens in the get schedule view frame.
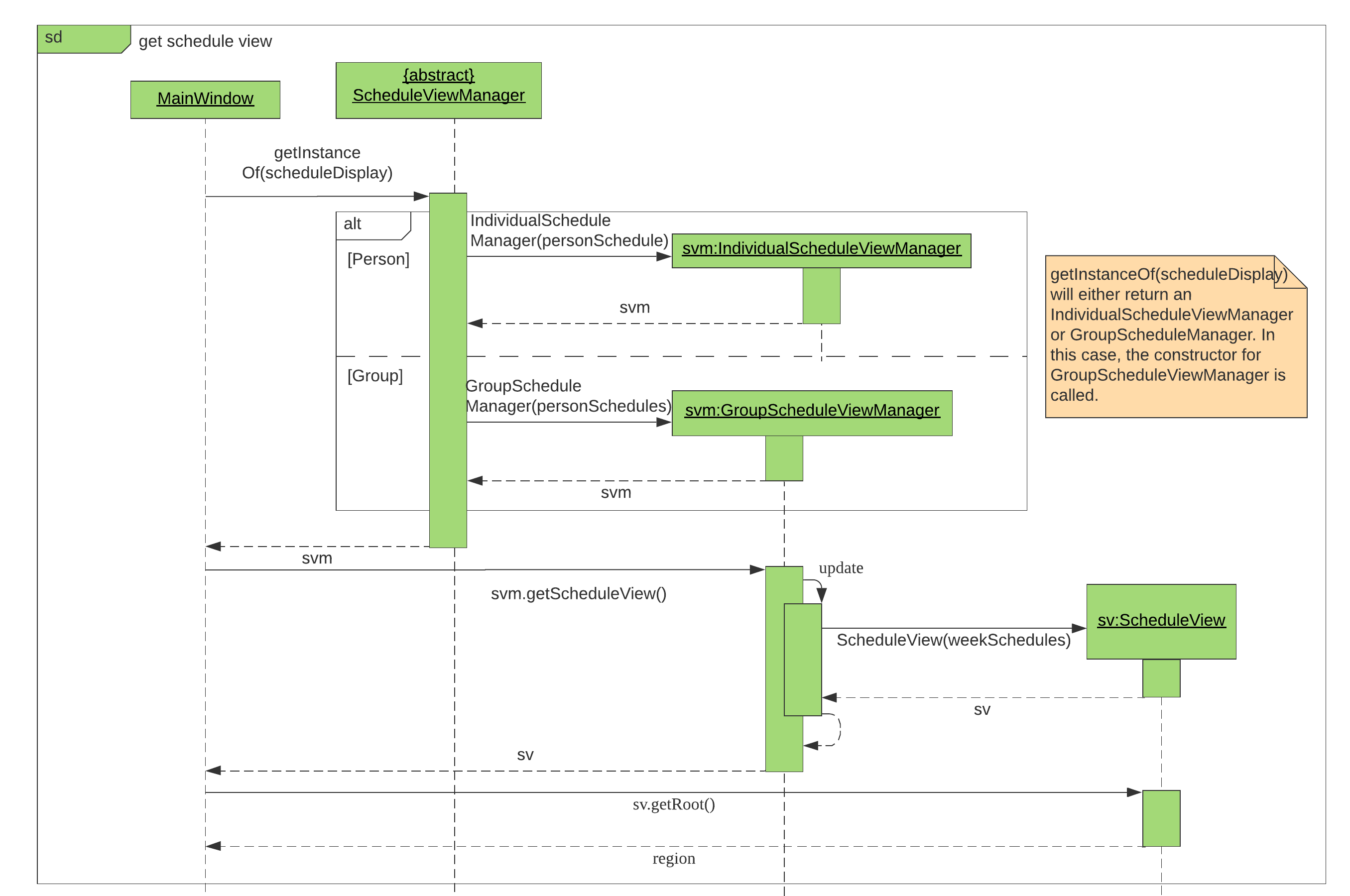
Details of how the graphics are created within the ScheduleView
have been described above and thus, are omitted in the diagram.
Design Considerations
Aspect: |
Choice |
Pros |
Cons |
Amount of detail present in schedule view. |
1. Enable users to see schedules up to 1 week in advance. |
1. Easy to implement. 2. Less likely for bugs when invoking other commands such as select and popup. |
1. Users may experience difficulty to plan meetings 2 or more weeks in advance. |
2. Enable users to see schedules up to 4 weeks in advance. (Current choice) |
1. Most users should be able to plan most of their meetings. |
1. Slightly more challenging to implement. 2. Slower as each request will take 4 times as long. |
|
2. Enable users to see schedules up to an indefinite weeks in advance. |
1. Every users should be able to plan their meetings. |
1. Slow requests as every query will regenerate a new set of graphics. |
|
We chose to allow users to see schedules up to 4 weeks in advance mainly due to usability. We recognise that most group meetings do not happen within a short period of 1 week as it may seem rushed for everyone in a group. We also found that it is unnecessary to enable users to see their schedules after the 1 month mark since it is most likely to not have been updated yet. Thus, showing schedules for up to 4 weeks should be sufficient for our design. |
Aspect: |
Choice |
Pros |
Cons |
Viewing some group member’s schedule in a group using the |
1. Filter, but do not recalculate the free time slot to the filtered group members from the command. (Current choice) |
1. Easier to implement.. 2. User can still keep track of the entire group’s schedule. |
1. Users may be misled to think that the |
2. filters, recalculate and display the common free time slot for the filtered members. |
1. There will not be any misleading empty blocks in a group’s schedule. |
1. Difficult to implement. 2. Each query will take a lot longer to process the locations data. |
|
We understand that users may want to inspect the schedules of some of his or her group members while still keeping track of the entire group’s common free time slots. This would be useful for users who want to organise partial group meetings with some of his or her group members before or after the official group meeting (where everyone attends). Furthermore, filtering a group member can easily be done by just creating a new group and adding group members to it. |